
Table of Contents
Introduction
In last tutorial I have given details on What is Variable Type and How Variable Types Work in TypeScript?
Now In this tutorial I am going to give you details on what are the difference variable types we have in TypeScript.
What are the Types Available In TypeScript
We have few different types in TypeScript, here are the list of all available types in TypeScript:
- number
- boolean
- string
- any
- array of number, string or any
- Tuple
- enum
- null
- Undefined
Next I will give you details on each type one by one and how you can use type annotation.
number
We have number, it can include any different number or floating point numbers.
For example:
let count: number;
count = 1;
count = 1.00;
boolean
We have boolean, it can stores the value which can be true or false
Example:
let validUser: boolean;
validUser = true;
validUser = false;
string
We have string type, it can store any string as showing below:
let siteName: string;
siteName = 'iTech Empires';
any
We have any which can store any values into the variable, like string, number, boolean.
let count: any;
count = 1;
count = 'abc';
count = true;
array
Array is basically a collection of multiple items, let’s say you want store different numbers in a variable or string.
Example of using Array in TypeScript:
let numbers: number[];
numbers = [1, 2, 3, 4, 5, 6];
As you can see in the above example we have a number variable with type of number and look at the end of the type we have pair of square brackets, this is how we declare array type in TypeScript.
Also optionally we can by default assign values to the array while defining as showing in below example:
let numbers: number[1, 2, 3, 4, 5];
Now let’s see how we can define different types of arrays in Typescript.
Few different Array Types examples from TypeScript:
// Array of number
let numbers: number[];
numbers = [1, 2, 3, 4, 5, 6];
// Array of String
let items: string[];
items = ['a', 'b', 'c', 'd'];
// Array of Any
let allItems: any[];
allItems = [1, 'a', true, 2.3];
As you can see into the above example we can set array of number, string and any.
You see that in allItems array with the type of any we can store any type of values inside this array however keep in mind that it is not a good practice to store different values in to this array unless you want to store values intentionally.
tuple
Tuple is a data type that allows you to create an array where the type of a fixed number of elements are known but need not be same.
When you access an element of a Tuple with a valid known index, the data of correct type will be returned.
In case you access an element outside the set of known indices, a union type will be used. Consider the following code snippet as an example:
// correct example
let person: [string, number] = ['Empire', 2018];
// wrong example
let person: [string, number] = [2018, 'Empire'];
// correct example
let person: [string, number] = ['Empire', 2018, 'Tech'];
// correct example
let person: [string, number] = ['Empire', 2018, 21];
// wrong example
let person: [string, number] = ['Empire', 2018, true];
enum
Next we have this enum type in TypeScript and this is my favourite type in typescript.
Let me give you example to explain how enum work in typescript and how it helps to avoid lot of complications
Let’s say we are working with group of related constant variables like colors, as showing below:
const ColorRed = 0;
const ColorGreen = 1;
const ColorBlue = 2;
If you notice the above code is little bit verbose right?
In a lot of Object Oriented Languages we have concept call enum and it’s we have it here as well in TypeScript.
So to avoid above issue, we put all of this related constant variables in a container called enum.
In typeScript we can declare enum like showing below:
enum Color {Red, Green, Blue};
And then you can use the above enum like this:
let backgroundColor = Color.Red;
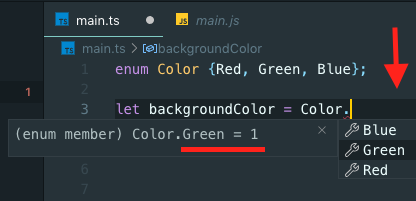
Now you can that we have IntelliSense here, you can see that the tooltip you see here allows us to complete this code without remembering all the details.
This is one of the thing is good about TypeScript and this one of great advantage of using type annotation in typescript.
One more thing if you notice here, have not set values to the Red, Green, Blue variables from the enum but they are still getting default values starting from 0 and increment by one for the next variable.
So basically the first element from the enum array is automatically get the value 0 and each subsequent element get’s an incremented value.
You don’t have explicitly sets those values but as a best practice it is better do so because chances are sometime in the future someone may work on your code so it’s better for this his understandings, basically in simple words you code will be more readable and maintainable.
For Example:
enum Color {
Red = 0,
Green = 1,
Blue = 2
}
Now let me show that how we get enum in javaScript to do that let’s compile the above using TypeScript compiler.
tsc main.ts
If you are not sure how to use typescript compiler or how to install in your system you get those details from the this tutorial – How to install TypeScript and Write TypeScript Program
So here is how we will get the enum into Javascript, look at the below code so this is how we implement the concept of enum in JavaScript.
var Color;
(function (Color) {
Color[Color["Red"] = 0] = "Red";
Color[Color["Green"] = 1] = "Green";
Color[Color["Blue"] = 2] = "Blue";
})(Color || (Color = {}));
var backgroundColor = Color.Red;
You can see it is very complicated to write enum in JavaScript but if you compare this to the TypeScript it is much cleaner and easy understand and remember.
null
You can declare a variable of type null by using the null keyword and can assign only null value to it. null is a subtype of all other types, you can assign it to a number or a boolean value.
As showing below Example:
let nullValue: null = null;
let numericValue: number = null;
undefined
You can use the undefined keyword as a data type to store the value undefined to it. As undefined is a subtype of all other types, just like null, you can assign it to a number or a boolean value. Refer below code example:
let undefinedValue: undefined = undefined;
let numericValue: number = undefined;