
Table of Contents
Introduction
In last tutorial I have given details on How to declare variables in TypeScript and What is the difference between Let and Var Keyword.
In this tutorial let’s see what is exactly Types In type Script and How they work?
What is Type in TypeScript and How they Work?
I am going to explain this by giving you an example, so I Am going to declare a variable called count and set it’s value to 1 and in the next line am going to try assigning a string to the same variable, let’s see what happens.
let count = 1;
count = 'abc';
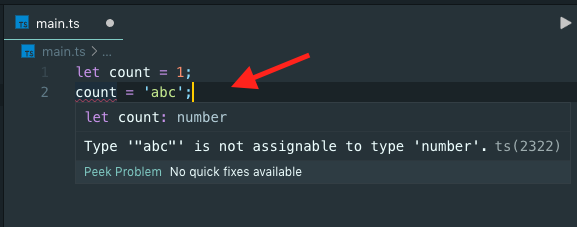
You see in the above screen that If I do that I immediately get compilation error message here telling me that “Type ‘”abc”‘ is not assignable to type ‘number'” meaning that this “abc” string is not assignable to type number, because the count variable is the type of number.
However quick note here, changing types of variable on fly is available in JavaScript but in typescript we cannot do that, it is not a good practice.
Okay so let’s take another example, Now here I am going to declare a variable whiteout initialising and then next I am going to assign different types values from the next line.
let count;
count = 1;
count = 'abc';
count = true;

Here if you hover your mouse on count variable you see that the type of this is now any meaning it can store any values like number, string, boolean etc.
As you can see in the code here I am able to set all this kind values to the variable and even TypeScript is accepting, it is not showing any compilation error.
In TypeScript if you define variable without initialising of settings it’s type by default it will set to any
So What is the solution to this?
If you don’t know value of the variable ahead of the time that’s when we use Type annotation.
Use type annotation
First do you know what is type annotation in TypeScript?
Type annotation is basically is way of defining type of a particular variable before using it.Very Simple Right?
So let’s fix the above problem using type annotation, see below example:
let count: number;
count = 1;
count = 'abc';
count = true;
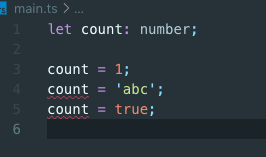
So here I am setting the type of count variable to number using colon.
And you see that on the fourth and fifth line we have got compilation error.
Conclusion
So what is the take away here is alway define a variable type in TypeScript, in that way typescript will show compilation error if we are going to mess with the variable type in further script, it helps to avoid issues in the application.