
In last tutorial I have given details on how you can create React application using Create React App package.
Must Learn – Ultimate way create your First React Application
Now in this tutorial I want to keep using the same application created from last tutorial but with little tricks, here we will delete all the files from src folder and create from scratch.
The goal is to give you details understanding on how React Virtual DOM exactly works.
If you have not read the last tutorial then I would suggest to follow that one before reading this tutorial.
Table of Contents
Delete all the files from SRC folder
As I told you I want you to delete all the files from src folder, so go ahead and delete following selected files:
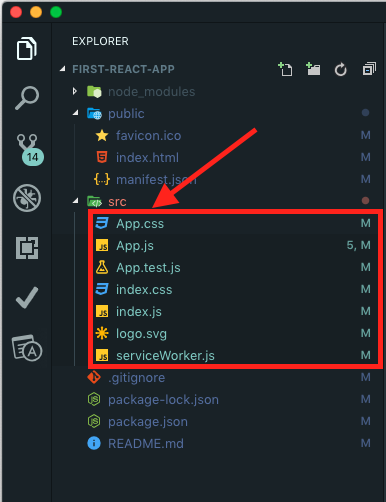
Run npm start
Navigate to your project directory from terminal or command prompt if you are on windows and execute following command:
npm start
You don’t have to worry about the output for now because the page will be blank because nothing is there right now.
Add new index.js File
Now let’s start by adding a new file in src folder calling it index.js
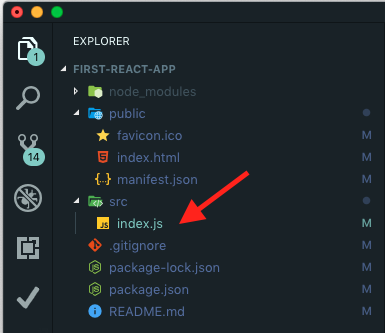
Next open up index.js file and in this file we need import couple of objects from React Module, if you not familiar with ES6 modules don’t worry just code along as showing below:
import React from 'react';
import ReactDOM from 'react-dom';
const element = <h1>Hello World</h1>;
console.log(element);
So in the above script we are importing React object from react module and similar we are importing ReactDOM object from react-dom module.
Then next we defining new element using const, const is the new feature from modern javascript previously we were only using var but now we have const and let. so for element we seeding an JSX expression, as I told you before in tutorial that Babel will compile this down to make it work on browser.
In to then last line we are just logging element into the console.
Note – there is one thing you need to know about the projects that are created using create-react-app package when you save the changes and if npm start is running on your terminal this application is automatically going to restarted now you don’t have go browser and refresh it, this is what we called hot module reloading.
So save the change and back to the browser and open up the console we can do that by ALT + Command + i on mac or ALT+CTL+I on windows or if that shortcut doest not work for you then goto the view menu on the top and Developer – Developer Tools
After opening the console you see following output , that is the output from JSX expression.
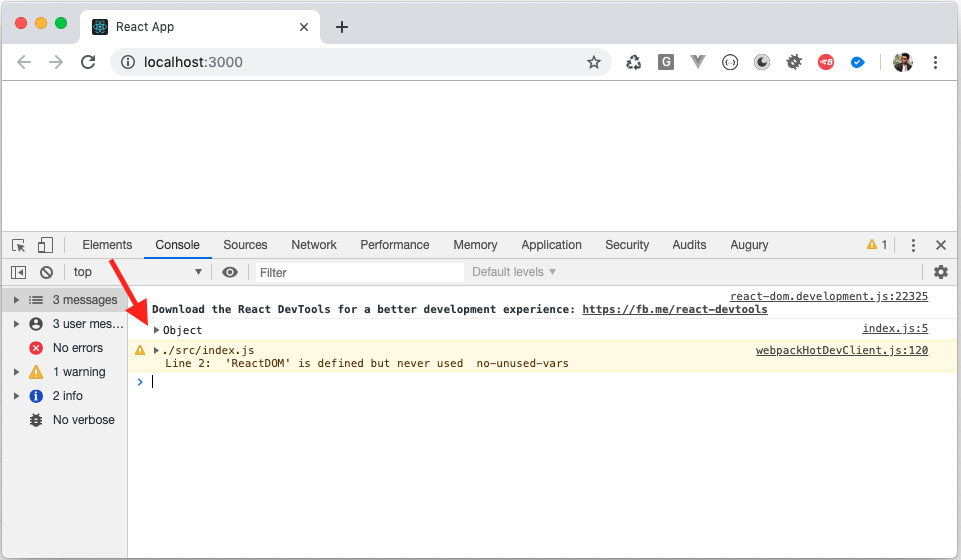
For example the object you see in console, it is the type of this element the type property is set to h1 and we have other bunch properties so earlier on the first tutorial on React series What is React and React Components? I talked about Virtual DOM which is light wight in memory representation of the UI it is not the real browser DOM is the virtual Document Object Model.
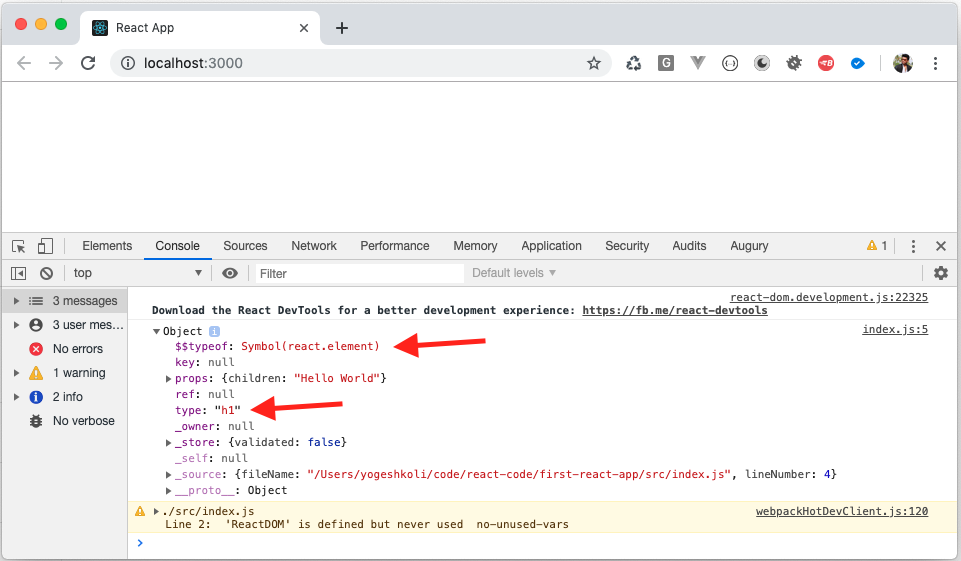
And this is object you see in the console is actually a Rect Element it is a part of Virtual DOM so whenever the state of this object changes React will get new React element then React will compare this element with the previous one it will figure out what is the change and then it will reach out to the real DOM and will get updated accordingly.
Render React Element to DOM
So in index.js we have JSX expression the result of that is React element which is the part of the Virtual DOM now we want to render this inside of the real DOM and if you notice that’s why we needs to import ReactDOM from the react-dom module on the top.
So let’s make some changes to use ReactDOM and ReactDOM to render element into real DOM.
import React from 'react';
import ReactDOM from 'react-dom';
const element = <h1>Hello World</h1>;
ReactDOM.render(element, document.getElementById('root'));
So we are calling React DOM and calling render function and as the first argument we need to pass element that we need to render and as a second argument we need to specify where in the real DOM we want to render this element. if you go back to the public/index.html file you will see that it has div with the id root
<div id="root"></div>
I told that this the container of our react application so we are using that here.
So save changes and go back to the browser and you see Hello World message, make sure npm start is still running.
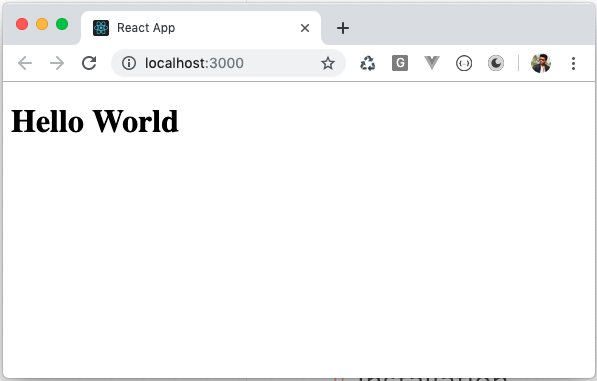
if you inspect in the developer tool, you see that the our div with the id root and inside of this div we have our react element
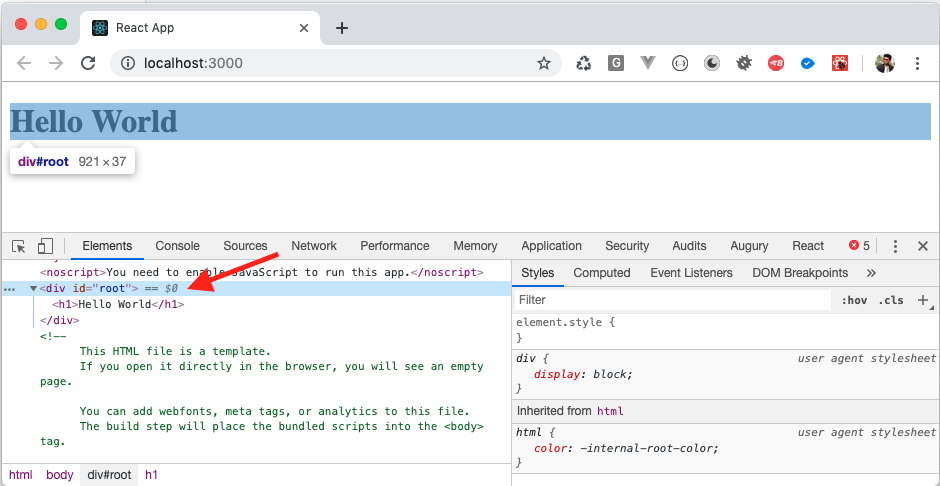
This is a very simple application just for demonstration and undertaking core process.
In a real word application in stead rendering a simple element in DOM, we render our App component.
App component is the root component of the React Application and it can include children components like menu, navigation, sidebar and profile etc.
So in React we always have tree of compontaend which will eventually produce the complex markup inside Real DOM.