
Table of Contents
Introduction:
In this tutorial I am going to provide a solution on how to create dynamic database tables along with dynamic fields in Laravel 5 project without writing a single SQL query. if you are Laravel developer and used migrations before you must pretty much familiar with this approach, Laravel migrations are incredibly simple and quicker way to interact with database tables here we will use same solution but run time and by that mean it can be a anything where you need dynamic table created in backend according to user need.
This solution can be applied to form builder projects backend, just like google forms where user can create dynamic forms so you always needs to deal with dynamic fields for the tables fields could be anything and any count so this eventually this tutorial will help you to handle backend operation of form builder.
Okay let’s dive into the solution, first think you need to be ready with Laravel project along with database configuration setting ready and yes you can use your existing project as well.
Solution:
If you have Laravel 5 project up and running then execute following command to create new controller into your project, we will use this controller to perform table creation operations:
$ php artisan make:controller TableController
Add following dependencies at top of the controller:
use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint;
Add this method into TableController, this is the method which is going to handle create operation for the table, you just needs to provide your table name and dynamic fields:
/app/Http/Controllers/TableController.php:
/** * Create dynamic table along with dynamic fields * * @param $table_name * @param array $fields * * @return \Illuminate\Http\JsonResponse */ public function createTable($table_name, $fields = []) { // check if table is not already exists if (!Schema::hasTable($table_name)) { Schema::create($table_name, function (Blueprint $table) use ($fields, $table_name) { $table->increments('id'); if (count($fields) > 0) { foreach ($fields as $field) { $table->{$field['type']}($field['name']); } } $table->timestamps(); }); return response()->json(['message' => 'Given table has been successfully created!'], 200); } return response()->json(['message' => 'Given table is already existis.'], 400); }
and now you can easily call above method like example showing below:
public function operate() { // set dynamic table name according to your requirements $table_name = 'demo'; // set your dynamic fields (you can fetch this data from database this is just an example) $fields = [ ['name' => 'field_1', 'type' => 'string'], ['name' => 'field_2', 'type' => 'text'], ['name' => 'field_3', 'type' => 'integer'], ['name' => 'field_4', 'type' => 'longText'] ]; return $this->createTable($table_name, $fields); }
Note: The above example is just simple for the demonstration you can update this example according to your needs and make fields dynamic and also we are setting up `id` as primary key auto increment for the table.
To remove the table from database use following method:
/** * To delete the tabel from the database * * @param $table_name * * @return bool */ public function removeTable($table_name) { Schema::dropIfExists($table_name); return true; }
Testing:
To test above solution I have created simple route to access operate() method from the controller showing below:
/routes/web.php:
Route::get('/create-table', 'TableController@operate');
Now we can access /create-table route and see what comes into place.
Here is the Result:
{ "message": "Given table has been successfully created!" }
And checkout the table from database:
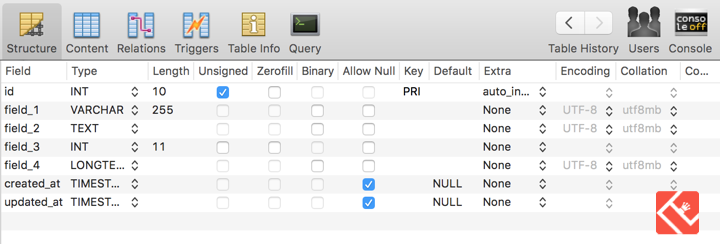
We are done.
If you find this solution helpful or have any question in mind let me know using comment box below.
that snippet is really useful for me
Thanks a lot
thank you for very good content.
but
How do I update the table? or add column in table
How to make soft delete (deleted_at) for dynamic created tables (without using Models)?
Sorry for my english/
Thank you!
Exactly what I was looking for. Thanks a lot. You save my day. but I wonder how can I create relation and retrieve relation from dynamic table?
Cool, but how would you add indexes such as unique(), atttibutes such as nullable(), and/or set sizes if needed.
Hi sir, i followed ur code and made some changes for creating dynamic table to dynamic users(i have passed the table name as array). But only 1 table is created. I need to create it for n number of users at the same time. Is that possible?
here’s modified code:
$table_name) {
if(!Schema:: hasTable($table_name)){
Schema::create($table_name, function(Blueprint $table) use ($fields,$table_name){
$table->increments(‘id’);
if(count($fields)>0){
foreach ($fields as $key => $field) {
$table->{$field[‘type’]}($field[‘name’]);
}
}
$table->timestamps();
});
return response()->json([$table_name.’ table has been created successfully!’],200);
}
return response()->json([‘table already exists’],400);
}
}
public function operate()
{
$username = [‘0’=>’cedric’,’1’=>’archie’,’2’=>’charlie’,’3’=>’bella’,’4’=>’alastor’];
foreach ($username as $key => $value) {
$fields = [
[‘name’ => ‘field_1’, ‘type’ => ‘string’],
[‘name’ => ‘field_2’, ‘type’ => ‘text’],
[‘name’ => ‘field_3’, ‘type’ => ‘integer’],
[‘name’ => ‘field_4’, ‘type’ => ‘longText’]
];
return $this->createTable($username, $fields);
}
}
}
Thanks for sharing knowledge.